Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications
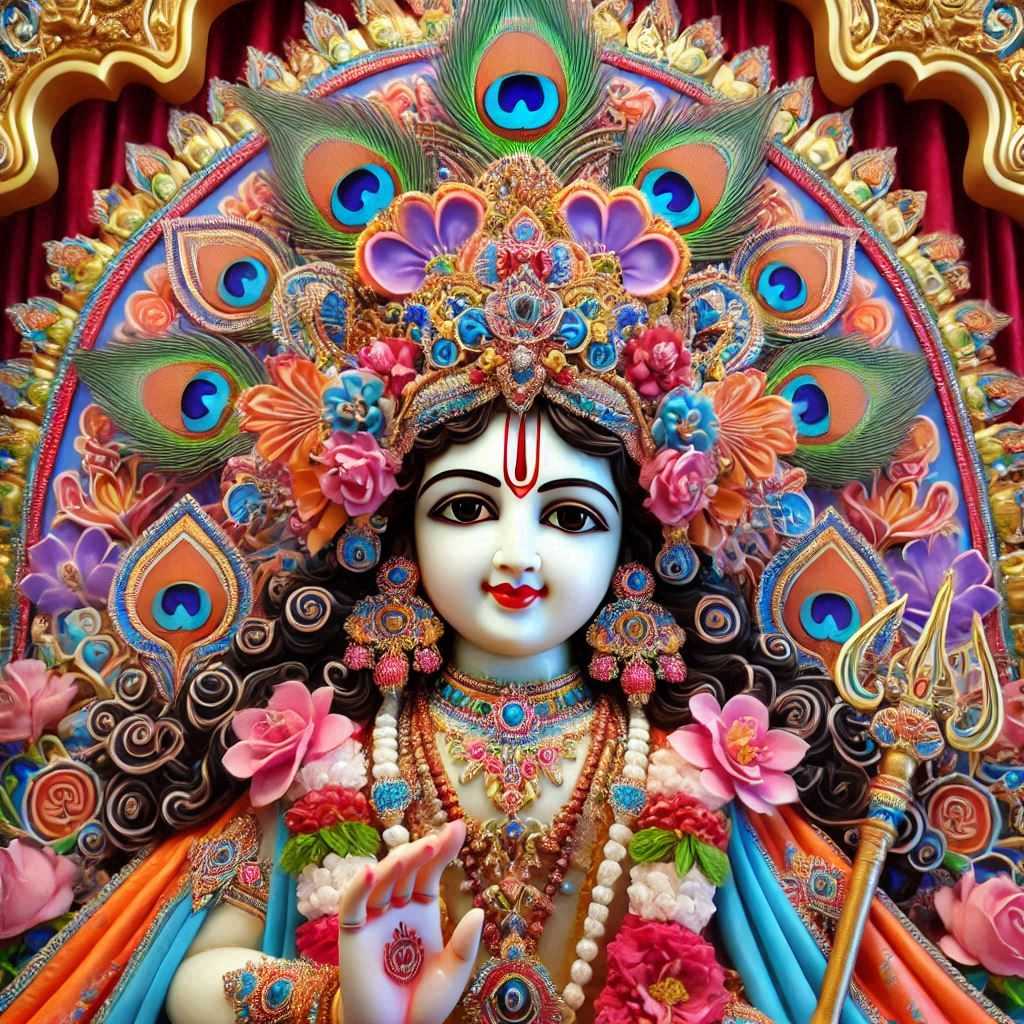
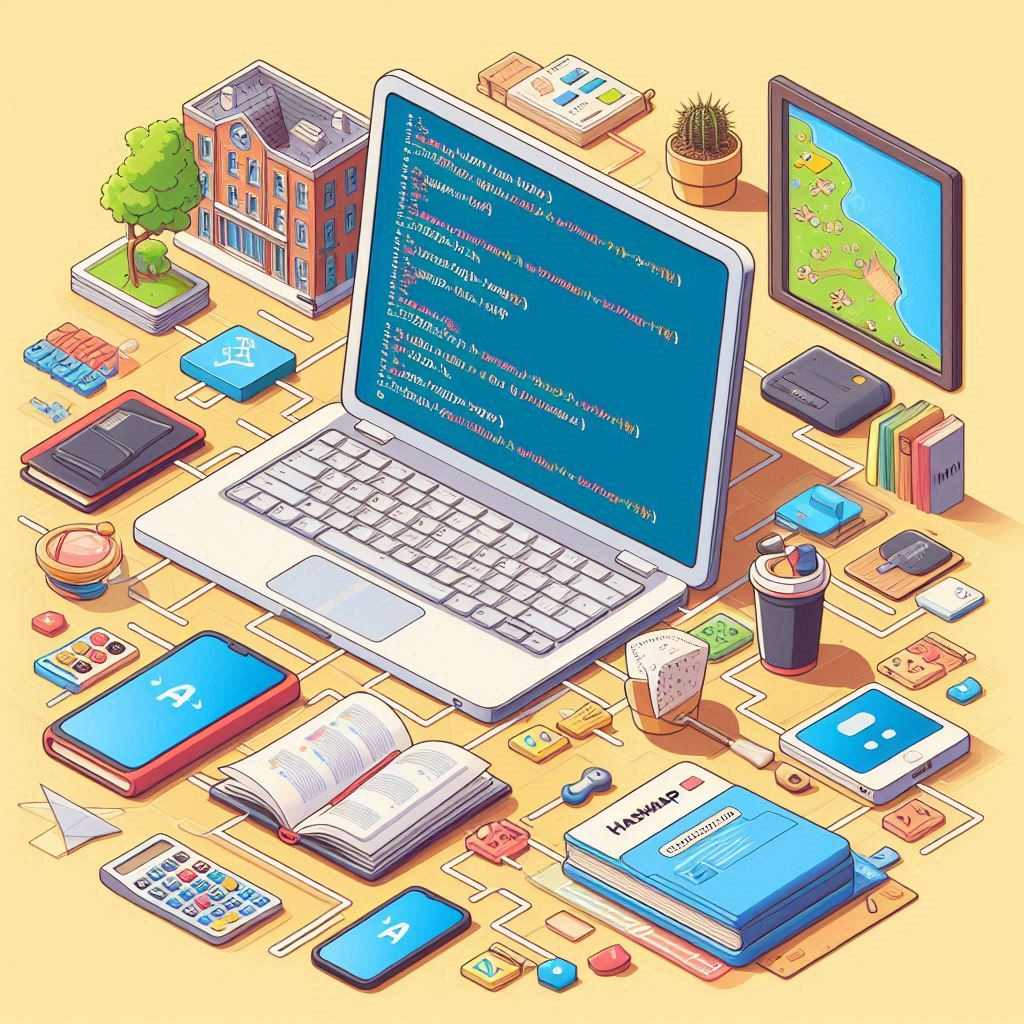
The Map
interface in Java is part of the java.util
package and is one of the most essential components of the Java Collections Framework. It is used to store and manage data in key-value pairs, making it a powerful data structure for various use cases.
In this blog, we will dive deep into the Map
interface, its features, operations, implementations, and real-world applications.
What is a Map in Java?
A Map
is a collection of objects that associates unique keys with corresponding values. Keys act as identifiers, while values hold the data. Each key in a Map
must be unique, but values can be duplicated.
Key Characteristics of Map
- Key-Value Pair Storage: Data is stored as
(key, value)
pairs. - Unique Keys: Duplicate keys are not allowed, but multiple keys can map to the same value.
- Non-Indexed: Unlike lists or arrays,
Map
does not use an index to access elements. - Null Handling: Certain implementations allow
null
keys and/or values. - Not Synchronized: Most implementations are not thread-safe unless explicitly stated.
Common Operations on Maps
The Map
interface provides a variety of methods for data manipulation. Here are some core operations:
Method | Description |
---|---|
put(K key, V value) |
Inserts or updates a key-value pair. |
get(Object key) |
Retrieves the value associated with the given key. |
remove(Object key) |
Removes the key-value pair for the specified key. |
containsKey(Object key) |
Checks if the map contains the given key. |
containsValue(Object value) |
Checks if the map contains the given value. |
keySet() |
Returns a set of all keys. |
values() |
Returns a collection of all values. |
entrySet() |
Returns a set of all key-value pairs (Map.Entry objects). |
Basic Example
import java.util.*;
public class MapExample {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(1, "One");
map.put(2, "Two");
map.put(3, "Three");
System.out.println("Keys: " + map.keySet()); // [1, 2, 3]
System.out.println("Values: " + map.values()); // [One, Two, Three]
System.out.println("Entry Set: " + map.entrySet()); // [1=One, 2=Two, 3=Three]
System.out.println("Get key 2: " + map.get(2)); // Two
map.remove(3);
System.out.println("After removal: " + map); // {1=One, 2=Two}
}
}
Output:
Keys: [1, 2, 3]
Values: [One, Two, Three]
Entry Set: [1=One, 2=Two, 3=Three]
Get key 2: Two
After removal: {1=One, 2=Two}
Implementations of Map
The Map
interface has several implementations, each designed for specific scenarios. Let’s explore them in detail.
1. HashMap
-
Overview: The
HashMap
is a widely used implementation of theMap
interface that uses a hash table to store key-value pairs. -
Key Features:
- No Order: The elements are not stored in any specific order.
- Allows Nulls: Allows one
null
key and multiplenull
values. - Fast: Provides constant-time performance for basic operations on average.
- Not Thread-Safe: Use
ConcurrentHashMap
orCollections.synchronizedMap()
for thread safety.
-
Example:
import java.util.HashMap; public class HashMapExample { public static void main(String[] args) { HashMap<Integer, String> hashMap = new HashMap<>(); hashMap.put(1, "One"); hashMap.put(2, "Two"); hashMap.put(null, "Three"); hashMap.put(4, null); System.out.println("HashMap: " + hashMap); } }
Output:
HashMap: {null=Three, 1=One, 2=Two, 4=null}
2. LinkedHashMap
-
Overview: Extends
HashMap
and maintains the insertion order of keys. -
Key Features:
- Preserves Order: Iterates in the order the keys were added.
- Allows Nulls: Similar to
HashMap
, allows onenull
key and multiplenull
values. - Slightly Slower: The overhead of maintaining order makes it slower than
HashMap
.
-
Example:
import java.util.LinkedHashMap; public class LinkedHashMapExample { public static void main(String[] args) { LinkedHashMap<Integer, String> linkedHashMap = new LinkedHashMap<>(); linkedHashMap.put(1, "One"); linkedHashMap.put(2, "Two"); linkedHashMap.put(3, "Three"); System.out.println("LinkedHashMap: " + linkedHashMap); } }
Output:
LinkedHashMap: {1=One, 2=Two, 3=Three}
3. TreeMap
-
Overview: A
TreeMap
is implemented as a Red-Black tree, storing keys in a sorted order. -
Key Features:
- Sorted Order: Keys are sorted either by their natural order or a custom comparator.
- No Null Keys: Does not allow
null
keys, butnull
values are permitted. - Performance: Operations like
put
andget
take O(log n) time.
-
Example:
import java.util.TreeMap; public class TreeMapExample { public static void main(String[] args) { TreeMap<String, Integer> treeMap = new TreeMap<>(); treeMap.put("Banana", 1); treeMap.put("Apple", 2); treeMap.put("Cherry", 3); System.out.println("TreeMap (sorted): " + treeMap); } }
Output:
TreeMap (sorted): {Apple=2, Banana=1, Cherry=3}
4. Hashtable
-
Overview: A legacy, synchronized implementation of the
Map
interface. -
Key Features:
- Thread-Safe: All methods are synchronized, making it suitable for multi-threaded environments.
- No Nulls: Does not allow
null
keys or values. - Slower: Due to synchronization, it is slower than
HashMap
.
-
Example:
import java.util.Hashtable; public class HashtableExample { public static void main(String[] args) { Hashtable<Integer, String> hashtable = new Hashtable<>(); hashtable.put(1, "One"); hashtable.put(2, "Two"); System.out.println("Hashtable: " + hashtable); } }
Output:
Hashtable: {1=One, 2=Two}
5. ConcurrentHashMap
-
Overview: A modern thread-safe
Map
implementation designed for high concurrency. -
Key Features:
- Thread-Safe: Allows multiple threads to read and write simultaneously without locking the entire map.
- No Nulls: Does not allow
null
keys or values. - Efficient: Outperforms
Hashtable
in multi-threaded environments.
-
Example:
import java.util.concurrent.ConcurrentHashMap; public class ConcurrentHashMapExample { public static void main(String[] args) { ConcurrentHashMap<Integer, String> concurrentMap = new ConcurrentHashMap<>(); concurrentMap.put(1, "One"); concurrentMap.put(2, "Two"); System.out.println("ConcurrentHashMap: " + concurrentMap); } }
Output:
ConcurrentHashMap: {1=One, 2=Two}
Real-World Applications
- Caching:
HashMap
orConcurrentHashMap
for storing frequently accessed data. - Configuration Management:
Properties
class for managing key-value pairs. - Sorted Data:
TreeMap
for maintaining natural or custom order.
Conclusion
The Map
interface in Java is a powerful and flexible data structure, crucial for handling collections of key-value pairs. Whether you need to store data in an unordered fashion, maintain the insertion order, or keep the keys sorted, Java offers various Map
implementations such as HashMap
, LinkedHashMap
, TreeMap
, and more, each tailored to specific requirements. By understanding the strengths and weaknesses of these implementations, you can optimize your application for performance, thread safety, and data organization. Mastering the Map
interface and its variants will undoubtedly enhance your problem-solving skills and improve the efficiency of your Java programs.
Comments
Blog Categories
Latest Blogs
- Thursday, January 16, 2025
A Complete Beginner’s Guide to Java Stream API with Detailed Examples. The Stream API in Java 8 simplifies data processin...
- Thursday, January 16, 2025
Java 8: A Comprehensive Guide with Examples and Use Cases. Java 8 brought a wave of powerful new features tha...
- Wednesday, January 15, 2025
An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples. Functional interfaces are an important concept in ...
- Wednesday, January 15, 2025
Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications. Spring Core provides the foundation for all other ...
- Wednesday, January 15, 2025
Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples. Java is a powerful and versatile programming langu...
- Monday, January 13, 2025
Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications. The Map interface in Java is a powerful and flexib...