Java 8: A Comprehensive Guide with Examples and Use Cases
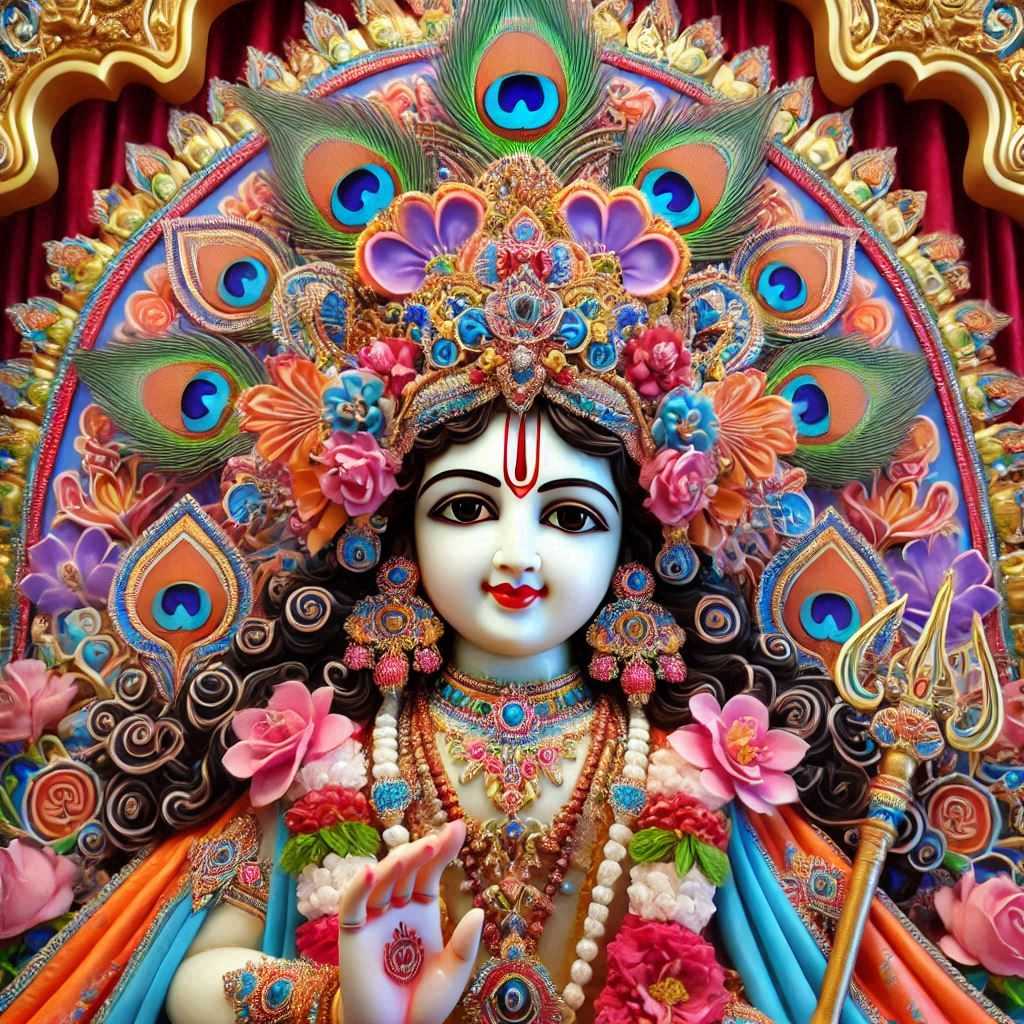
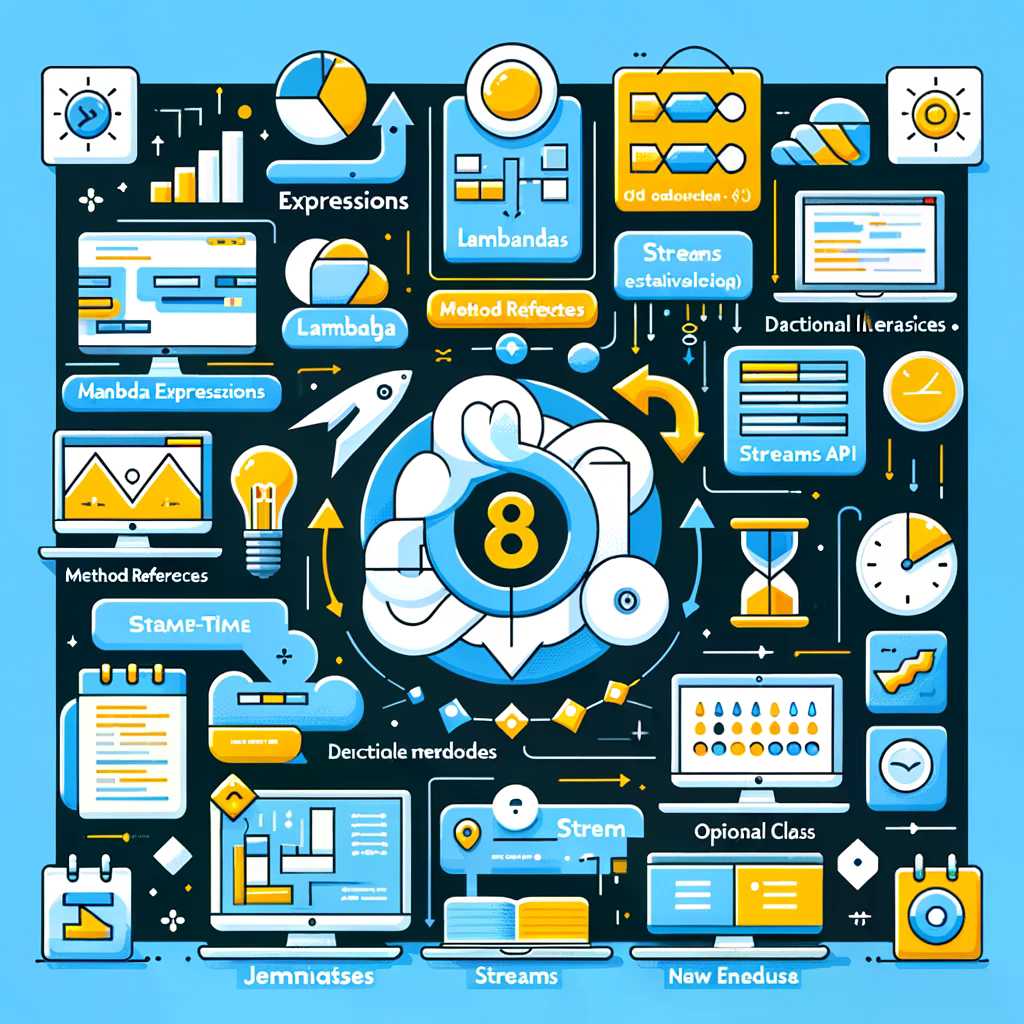
Java 8 is one of the most transformative updates in the history of Java. Released in March 2014, it introduced several key features that embraced the functional programming paradigm, made Java code more concise, and brought modern programming practices to the language. In this blog, we’ll explore Java 8 in-depth with examples, use cases, and practical applications of its major features.
1. Lambda Expressions
What are Lambda Expressions?
Lambda expressions allow you to define anonymous methods (functions) in a concise way. They provide a functional-style approach to Java programming, reducing boilerplate code for interfaces with a single abstract method (functional interfaces).
Syntax:
(parameters) -> expression
(parameters) -> { statements }
Example: Sorting with Lambda
Before Java 8:
List<String> names = Arrays.asList("John", "Jane", "Tom");
Collections.sort(names, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
Using Lambda in Java 8:
List<String> names = Arrays.asList("John", "Jane", "Tom");
Collections.sort(names, (s1, s2) -> s1.compareTo(s2));
Use Case:
Lambda expressions are ideal for scenarios where you need to implement small blocks of code for functional interfaces, such as event handling, sorting, or iterations.
2. Method References
What are Method References?
Method references are a shorthand notation for calling methods directly. They are syntactic sugar for lambda expressions when the lambda body simply calls a method.
Types of Method References:
-
Reference to a Static Method
Example:Function<String, Integer> parseInt = Integer::parseInt; System.out.println(parseInt.apply("123")); // Output: 123
-
Reference to an Instance Method of a Particular Object
Example:Consumer<String> printer = System.out::println; printer.accept("Hello, Method Reference!"); // Output: Hello, Method Reference!
-
Reference to an Instance Method of an Arbitrary Object
Example:List<String> names = Arrays.asList("John", "Jane"); names.forEach(System.out::println); // Prints each name
-
Reference to a Constructor
Example:Supplier<List<String>> listSupplier = ArrayList::new; List<String> list = listSupplier.get();
Use Case:
Method references make code more readable and concise, especially when working with the Streams API or functional interfaces.
3. Streams API
What is the Streams API?
The Streams API provides a functional-style way to process sequences of elements (like collections). It supports operations such as filtering, mapping, and reducing, which are processed lazily for better performance.
Example: Filter and Map with Streams
List<String> names = Arrays.asList("John", "Jane", "Tom", "Alice");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.map(String::toUpperCase)
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [JOHN, JANE]
Key Stream Operations:
- Intermediate Operations: Transform the stream (e.g.,
filter
,map
,sorted
). - Terminal Operations: Produce a result or side-effect (e.g.,
collect
,forEach
,reduce
).
Use Case:
The Streams API is perfect for:
- Processing large datasets.
- Writing clean and declarative code for data transformations.
4. Functional Interfaces
What are Functional Interfaces?
A functional interface is an interface with exactly one abstract method. Java 8 introduced many built-in functional interfaces in the java.util.function
package.
Key Functional Interfaces:
- Predicate: Takes a single argument and returns
boolean
. - Function<T, R>: Takes an input of type
T
and returns a result of typeR
. - Consumer: Takes a single argument and performs an operation but returns no result.
- Supplier: Provides a result without taking any input.
Example: Using Predicate
Predicate<Integer> isEven = number -> number % 2 == 0;
System.out.println(isEven.test(4)); // Output: true
System.out.println(isEven.test(5)); // Output: false
Use Case:
Functional interfaces simplify tasks like filtering, mapping, and applying operations on data.
5. Default and Static Methods in Interfaces
What are Default and Static Methods?
Before Java 8, interfaces could only have abstract methods. Java 8 introduced default and static methods, allowing interfaces to include method implementations.
Example: Default Method
interface Vehicle {
void drive();
default void honk() {
System.out.println("Honking...");
}
}
class Car implements Vehicle {
public void drive() {
System.out.println("Driving a car...");
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car();
car.drive();
car.honk(); // Output: Honking...
}
}
Use Case:
Default methods allow backward compatibility, enabling new methods to be added to existing interfaces without breaking implementations.
6. Optional Class
What is Optional?
The Optional class is a container for values that may or may not be null. It helps avoid NullPointerExceptions by providing methods to handle optional values gracefully.
Example: Using Optional
Optional<String> optionalName = Optional.ofNullable(null);
System.out.println(optionalName.orElse("Default Name")); // Output: Default Name
optionalName.ifPresent(name -> System.out.println("Name: " + name)); // Won't print anything
Use Case:
Use Optional
to handle null values safely, especially in APIs where null values are common.
7. New Date and Time API
What is the New Date and Time API?
Java 8 introduced the java.time
package, providing a more modern and thread-safe way to handle dates and times.
Example: LocalDate and LocalTime
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
public class DateTimeExample {
public static void main(String[] args) {
LocalDate date = LocalDate.now();
LocalTime time = LocalTime.now();
System.out.println("Current Date: " + date);
System.out.println("Current Time: " + time);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
System.out.println("Formatted Date: " + date.format(formatter));
}
}
Use Case:
The new API simplifies working with dates, times, and time zones, making it easier to perform date-related calculations and formatting.
8. Nashorn JavaScript Engine
The Nashorn engine allows you to execute JavaScript code directly in Java applications.
Example: Executing JavaScript
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
public class NashornExample {
public static void main(String[] args) throws Exception {
ScriptEngine engine = new ScriptEngineManager().getEngineByName("nashorn");
engine.eval("print('Hello from JavaScript!');");
}
}
Use Case:
Nashorn is useful for embedding scripting capabilities into Java applications.
Conclusion
Java 8 brought a wave of powerful new features that transformed the way developers write Java code. By embracing functional programming, introducing the Streams API, and modernizing key features like date and time handling, Java 8 made code more concise, expressive, and efficient.
Mastering these features is essential for any Java developer aiming to write clean, maintainable, and future-proof code. Whether you're working on data processing, APIs, or building scalable systems, Java 8 provides the tools to get the job done effectively.
If something seems incorrect or needs adjustment, please let me know!
Comments
Blog Categories
Latest Blogs
- Thursday, January 16, 2025
A Complete Beginner’s Guide to Java Stream API with Detailed Examples. The Stream API in Java 8 simplifies data processin...
- Thursday, January 16, 2025
Java 8: A Comprehensive Guide with Examples and Use Cases. Java 8 brought a wave of powerful new features tha...
- Wednesday, January 15, 2025
An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples. Functional interfaces are an important concept in ...
- Wednesday, January 15, 2025
Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications. Spring Core provides the foundation for all other ...
- Wednesday, January 15, 2025
Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples. Java is a powerful and versatile programming langu...
- Monday, January 13, 2025
Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications. The Map interface in Java is a powerful and flexib...