An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples
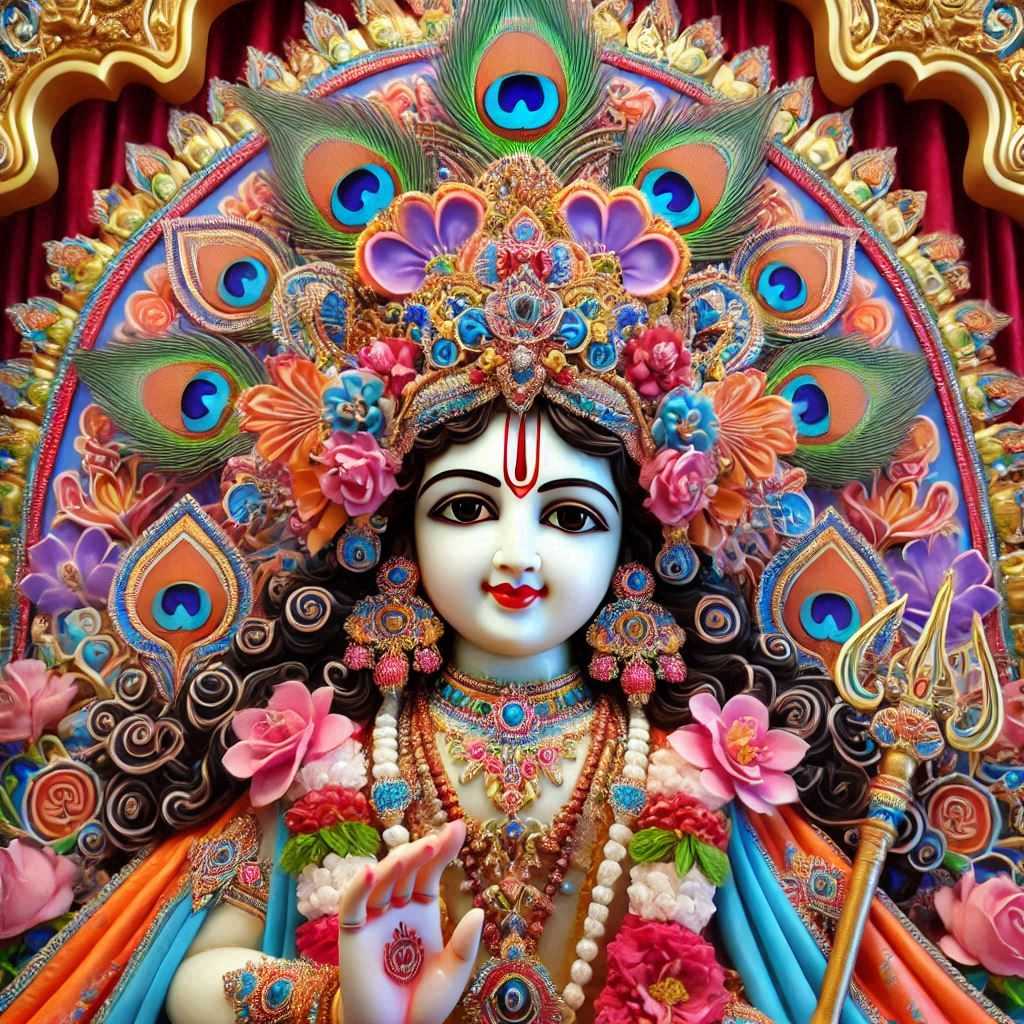
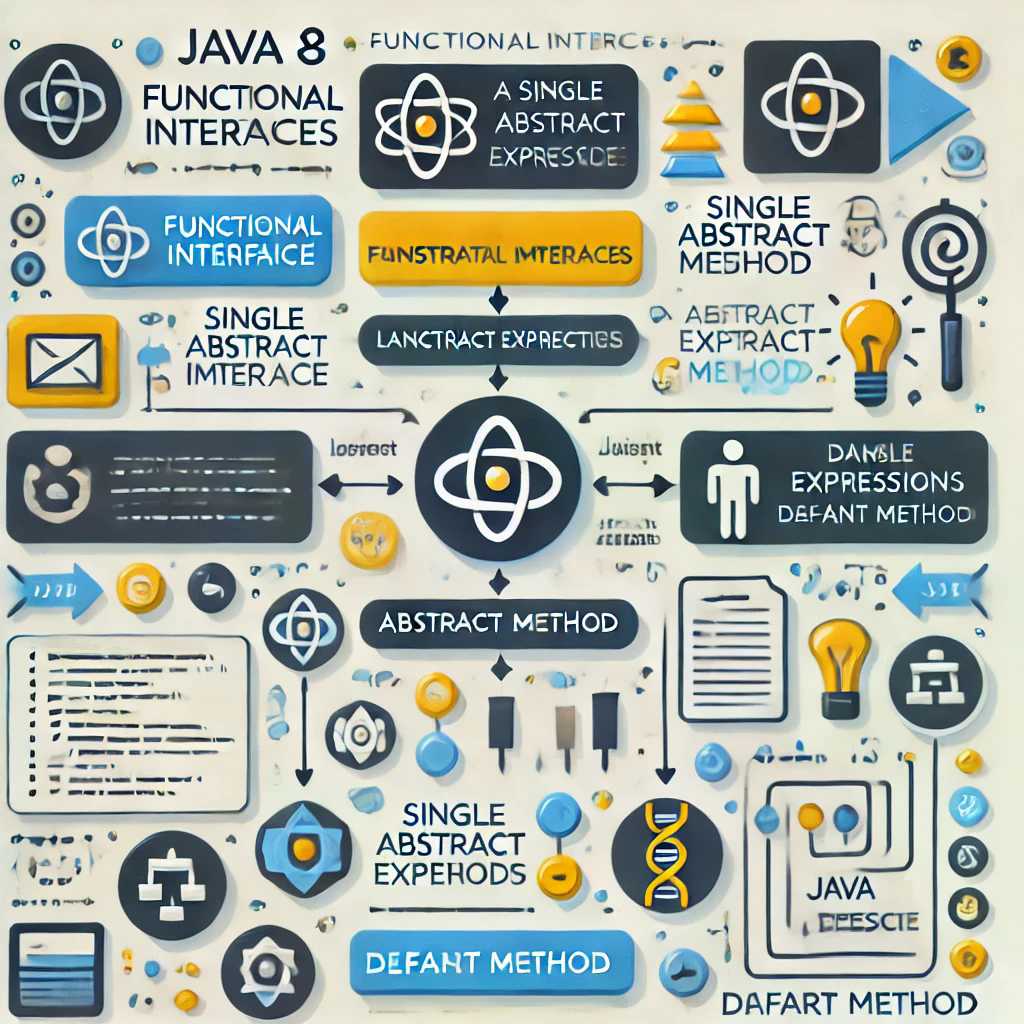
Introduction
In recent versions of Java, functional programming features have been introduced, making Java more powerful and expressive. One of the key elements of functional programming in Java is functional interfaces. If you're new to Java or just starting to learn about functional programming, this blog will walk you through what functional interfaces are, why they are useful, and how to use them with simple examples.
What is a Functional Interface?
A functional interface in Java is an interface that has only one abstract method. Think of it as an interface that describes a single behavior (or action) that can be performed. Functional interfaces are the foundation for lambda expressions in Java, which allow you to write more concise and readable code.
Key Points About Functional Interfaces:
- Single Abstract Method: A functional interface must have exactly one abstract method (i.e., a method without a body).
- Can Have Default and Static Methods: Although there should only be one abstract method, a functional interface can have multiple default methods (methods with a body) and static methods.
- Used with Lambda Expressions: Functional interfaces are often used with lambda expressions, which allow you to write code in a more compact form.
Example of a Functional Interface:
@FunctionalInterface
public interface MyFunctionalInterface {
void myMethod(); // Abstract method
// Default method
default void defaultMethod() {
System.out.println("This is a default method.");
}
// Static method
static void staticMethod() {
System.out.println("This is a static method.");
}
}
myMethod()
is the abstract method that makes this a functional interface.defaultMethod()
is a default method with a body.staticMethod()
is a static method, which is allowed in a functional interface.
Why Are Functional Interfaces Important?
Functional interfaces allow us to use lambda expressions in Java, which is a way to write more compact and readable code. Before Java 8, we would have to write full implementations for interfaces, which could make the code more complicated. With functional interfaces and lambda expressions, you can define simple behaviors in a single line.
Advantages of Functional Interfaces:
- Concise Code: With functional interfaces, you can write simple actions in a more compact way using lambda expressions.
- Easier to Read: Lambda expressions make the code more readable and expressive.
- Supports Functional Programming: They allow Java to embrace functional programming principles, which is a programming style where you treat actions as data (i.e., passing methods around as arguments).
How to Use a Functional Interface
A functional interface is typically used with a lambda expression. A lambda expression is a simple way to implement the abstract method of the functional interface in a more compact form.
Syntax of Lambda Expressions:
(parameters) -> expression
Example: Using a Functional Interface with a Lambda Expression
Let’s use the MyFunctionalInterface
example from earlier:
public class Main {
public static void main(String[] args) {
// Using lambda expression to implement MyFunctionalInterface
MyFunctionalInterface myFunc = () -> System.out.println("Lambda expression is invoked!");
myFunc.myMethod(); // Invokes the method defined in the functional interface
}
}
In this example:
- The lambda expression
() -> System.out.println("Lambda expression is invoked!")
is used to provide the implementation for the abstract methodmyMethod()
of the functional interface. - We then call
myMethod()
onmyFunc
, and it prints "Lambda expression is invoked!" to the console.
Common Built-In Functional Interfaces in Java
Java provides several common functional interfaces that can be used directly in your programs. These interfaces are part of the java.util.function
package and are frequently used in streams and other Java 8 features.
-
Function<T, R>: Represents a function that takes an input of type
T
and returns a result of typeR
.Function<Integer, String> intToString = i -> "Number: " + i; System.out.println(intToString.apply(5)); // Output: "Number: 5"
-
Predicate: Represents a condition that returns a boolean (true/false).
Predicate<Integer> isEven = i -> i % 2 == 0; System.out.println(isEven.test(4)); // Output: true
-
Consumer: Represents an action that takes an input of type
T
but does not return anything.Consumer<String> printMessage = message -> System.out.println(message); printMessage.accept("Hello, world!"); // Output: "Hello, world!"
-
Supplier: Represents a supplier that produces a result of type
T
but does not take any input.Supplier<Double> randomNumber = () -> Math.random(); System.out.println(randomNumber.get()); // Output: A random number between 0 and 1
-
UnaryOperator: A special case of
Function
where both the input and output types are the same.UnaryOperator<Integer> square = x -> x * x; System.out.println(square.apply(4)); // Output: 16
How to Create Your Own Functional Interface
You can create your own functional interfaces by following these steps:
- Declare an interface with one abstract method.
- Optionally, add default methods and static methods.
Example: Custom Functional Interface
@FunctionalInterface
public interface MyCalculator {
int calculate(int a, int b); // Single abstract method
// Default method
default String getOperation() {
return "Addition";
}
}
You can now use a lambda expression to implement the calculate()
method:
public class CalculatorTest {
public static void main(String[] args) {
// Lambda expression to implement calculate method
MyCalculator add = (a, b) -> a + b;
// Using the lambda expression
System.out.println(add.calculate(5, 3)); // Output: 8
}
}
In this example, the lambda expression (a, b) -> a + b
provides the implementation of the calculate()
method of MyCalculator
.
Using Functional Interfaces with Streams
Functional interfaces play a big role in Java's Stream API, which was introduced in Java 8. Streams allow you to perform operations like filtering, mapping, and reducing collections of data in a more functional way.
Example: Using a Functional Interface with Streams
import java.util.Arrays;
import java.util.List;
import java.util.function.Predicate;
public class StreamExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
// Predicate functional interface to check if a number is even
Predicate<Integer> isEven = num -> num % 2 == 0;
// Filter even numbers and print them
numbers.stream()
.filter(isEven)
.forEach(System.out::println); // Output: 2 4 6
}
}
In this example:
- We use the Predicate functional interface to define a condition (
isEven
). - We then use the
filter()
method in the Stream API to apply the predicate and print only the even numbers.
Conclusion
Functional interfaces are an important concept in Java, especially since the introduction of lambda expressions in Java 8. They allow you to write clean, concise, and readable code. Whether you're working with streams, writing custom functional interfaces, or simply simplifying your code with lambda expressions, understanding functional interfaces will help you take full advantage of Java’s functional programming features.
By using functional interfaces, you can make your Java applications more flexible, modular, and easier to maintain. If you're new to this concept, start by experimenting with simple examples, and you'll soon realize how much easier it makes coding in Java!
If something seems incorrect or needs adjustment, please let me know!
Comments
Blog Categories
Latest Blogs
- Thursday, January 16, 2025
A Complete Beginner’s Guide to Java Stream API with Detailed Examples. The Stream API in Java 8 simplifies data processin...
- Thursday, January 16, 2025
Java 8: A Comprehensive Guide with Examples and Use Cases. Java 8 brought a wave of powerful new features tha...
- Wednesday, January 15, 2025
An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples. Functional interfaces are an important concept in ...
- Wednesday, January 15, 2025
Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications. Spring Core provides the foundation for all other ...
- Wednesday, January 15, 2025
Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples. Java is a powerful and versatile programming langu...
- Monday, January 13, 2025
Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications. The Map interface in Java is a powerful and flexib...