Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications
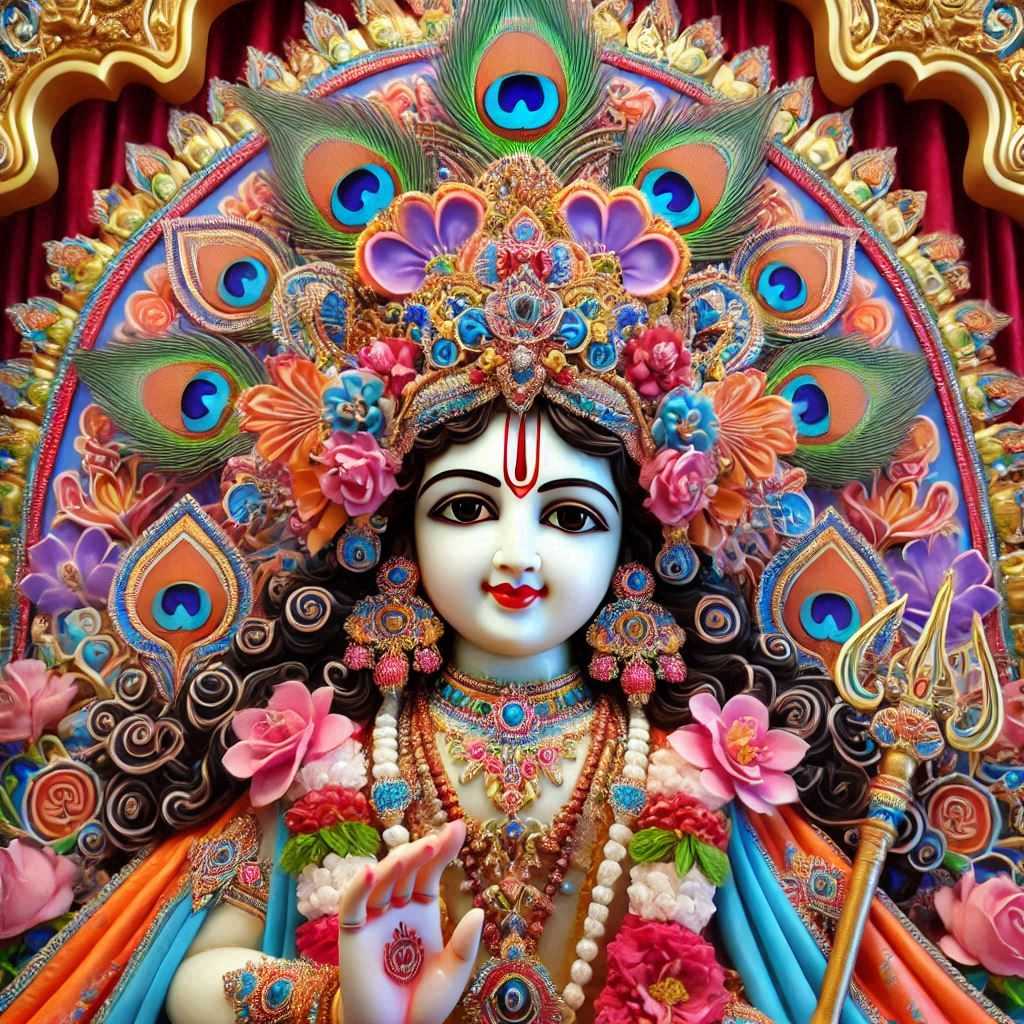
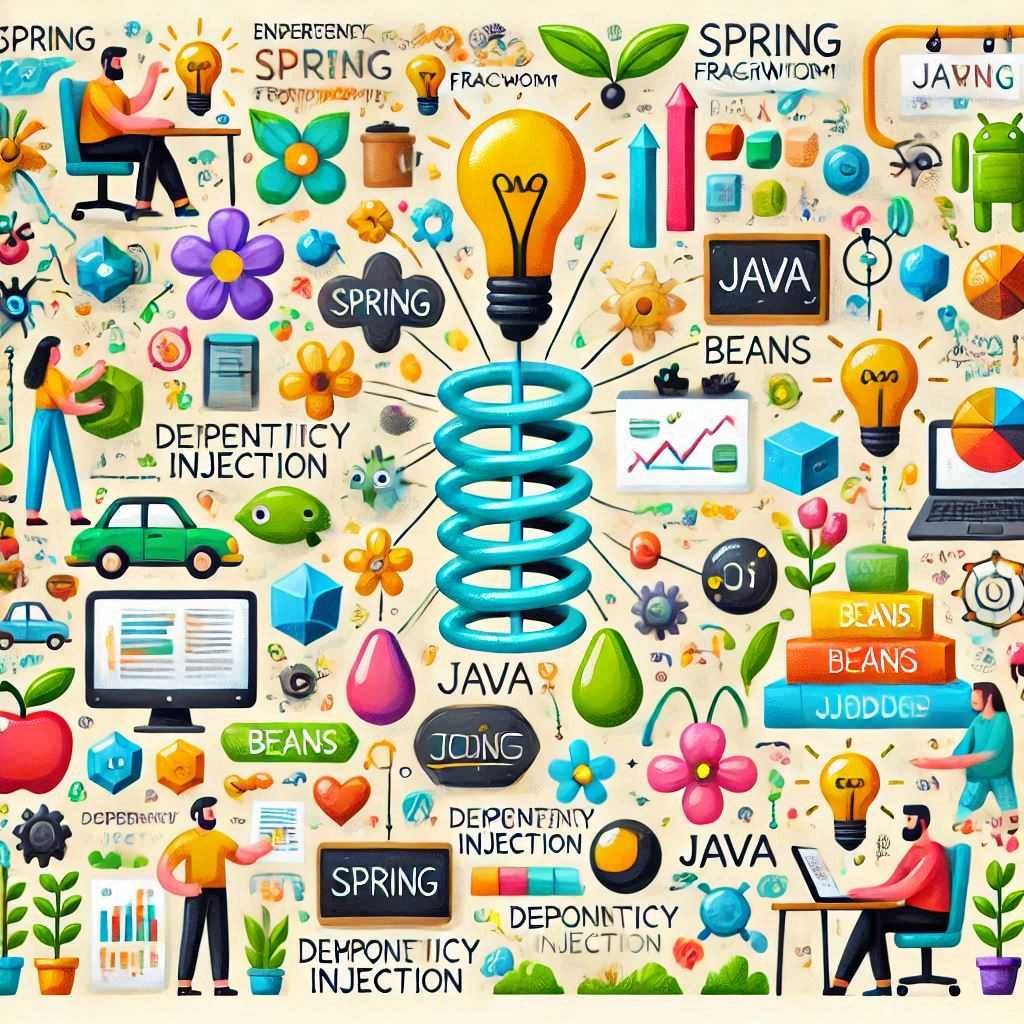
The Spring Framework is one of the most widely used frameworks in Java development due to its flexibility, modularity, and wide array of tools and features. At the heart of the Spring ecosystem is Spring Core, which forms the foundation for other Spring modules like Spring MVC, Spring Data, and Spring Security. This blog provides an in-depth look at the core concepts of Spring, explaining its fundamentals and how it enables developers to build maintainable, modular, and efficient Java applications.
1. What is Spring Core?
Spring Core is the fundamental part of the Spring Framework that deals with key concepts like Inversion of Control (IoC), Dependency Injection (DI), and Aspect-Oriented Programming (AOP). These core concepts help developers write loosely coupled, highly maintainable code.
Spring Core offers several key features:
- Bean Lifecycle Management: Spring manages the creation, configuration, and lifecycle of objects known as beans.
- Dependency Injection (DI): Spring's DI mechanism helps in managing dependencies between beans, allowing objects to be loosely coupled and easily tested.
- Aspect-Oriented Programming (AOP): Spring AOP provides a way to separate cross-cutting concerns (like logging, security, etc.) from business logic.
2. Inversion of Control (IoC)
Inversion of Control (IoC) is the principle that forms the backbone of the Spring Framework. IoC is a design principle where the control of object creation and dependency management is transferred from the application code to a container, often referred to as the Spring IoC container.
In a typical Java application, developers are responsible for creating and managing the objects themselves. In Spring, this responsibility is delegated to the container, which manages the lifecycle and dependencies of objects.
Key Components of IoC in Spring:
- ApplicationContext: This is the central interface to the Spring IoC container. It is responsible for managing the beans and their configurations.
- Beans: In Spring, an object is called a bean if it is created and managed by the Spring IoC container.
Example of IoC in Action:
public class Employee {
private String name;
private Department department;
// Getters and setters
}
public class Department {
private String name;
// Getters and setters
}
To manage the creation of these objects using IoC, you need to define beans in the Spring configuration file (XML or Java-based configuration).
Java-based Configuration:
@Configuration
public class AppConfig {
@Bean
public Employee employee() {
return new Employee("John Doe", department());
}
@Bean
public Department department() {
return new Department("Engineering");
}
}
Spring Boot Configuration:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
ApplicationContext context = SpringApplication.run(Application.class, args);
Employee employee = context.getBean(Employee.class);
System.out.println("Employee: " + employee.getName());
}
}
In this example, the Spring container creates the Employee
and Department
objects and injects the dependencies automatically.
3. Dependency Injection (DI)
Dependency Injection (DI) is one of the key features of Spring Core and is closely related to IoC. DI allows you to inject dependent objects into a class rather than creating them inside the class, which makes the code more modular and easier to test.
There are three types of Dependency Injection in Spring:
- Constructor Injection: Dependencies are provided through the class constructor.
- Setter Injection: Dependencies are provided through setter methods.
- Field Injection: Dependencies are provided directly to fields (less recommended due to tight coupling).
Constructor Injection:
public class Employee {
private Department department;
public Employee(Department department) {
this.department = department;
}
// Getter and setter
}
@Configuration
public class AppConfig {
@Bean
public Employee employee() {
return new Employee(department());
}
@Bean
public Department department() {
return new Department("Engineering");
}
}
Setter Injection:
public class Employee {
private Department department;
public void setDepartment(Department department) {
this.department = department;
}
// Getter
}
@Configuration
public class AppConfig {
@Bean
public Employee employee() {
Employee employee = new Employee();
employee.setDepartment(department());
return employee;
}
@Bean
public Department department() {
return new Department("Engineering");
}
}
In both examples, Spring automatically injects the Department
object into the Employee
class.
4. Bean Lifecycle in Spring
In Spring, beans are created and managed by the Spring IoC container. Understanding the bean lifecycle is crucial for managing the lifecycle of beans and resources in the application.
The lifecycle of a Spring bean can be broken down into several steps:
- Instantiation: The Spring container instantiates the bean.
- Dependency Injection: The container injects the required dependencies.
- Initialization: Spring calls any
@PostConstruct
methods or initialization methods specified in theInitializingBean
interface orinit-method
attribute in XML configuration. - Destruction: When the container is destroyed or the bean is no longer needed, Spring calls the
@PreDestroy
methods or methods specified in thedestroy-method
attribute in XML configuration.
Example: Bean Lifecycle in Spring
@Component
public class Employee {
@PostConstruct
public void init() {
System.out.println("Employee Bean Initialized");
}
@PreDestroy
public void cleanup() {
System.out.println("Employee Bean Destroyed");
}
}
Spring will call the init()
method after the bean is initialized and the cleanup()
method when the bean is destroyed.
5. Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) allows you to separate concerns that affect multiple classes, such as logging, transaction management, and security. In AOP, you define aspects (cross-cutting concerns) that can be applied to various points of execution in your application.
Key AOP Concepts:
- Aspect: A modularization of a concern that cuts across multiple classes (e.g., logging).
- Join Point: A point in the execution of the program where an aspect can be applied (e.g., method execution).
- Advice: The code that is executed at a particular join point.
- Pointcut: An expression that defines where advice should be applied.
Example: Using AOP in Spring
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Logging before method: " + joinPoint.getSignature().getName());
}
}
In this example, the @Before
annotation indicates that the logBefore()
method will be executed before any method in the com.example.service
package is invoked.
6. Spring Core in Action
Spring Core’s core features like IoC, DI, and AOP are often used in combination to build robust applications. By following best practices and using Spring Core's features, you can create clean, testable, and maintainable code.
Here’s a simple example of a Spring Core-based application that uses IoC and DI:
@Configuration
public class AppConfig {
@Bean
public Employee employee() {
return new Employee("John Doe", department());
}
@Bean
public Department department() {
return new Department("Engineering");
}
}
public class Application {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
Employee employee = context.getBean(Employee.class);
System.out.println(employee.getName() + " works in the " + employee.getDepartment().getName() + " department.");
}
}
This application defines beans for Employee
and Department
, injects dependencies using constructor injection, and outputs the employee's department.
Conclusion
Spring Core provides the foundation for all other Spring modules and is essential for building scalable, maintainable, and testable Java applications. By mastering key concepts such as Inversion of Control (IoC), Dependency Injection (DI), Aspect-Oriented Programming (AOP), and understanding the lifecycle of Spring beans, you can take full advantage of the power Spring offers. As you continue to build more complex applications, you will find that Spring Core simplifies the management of objects, configurations, and dependencies, leading to more efficient and maintainable code.
If something seems incorrect or needs adjustment, please let me know!
Comments
Blog Categories
Latest Blogs
- Thursday, January 16, 2025
A Complete Beginner’s Guide to Java Stream API with Detailed Examples. The Stream API in Java 8 simplifies data processin...
- Thursday, January 16, 2025
Java 8: A Comprehensive Guide with Examples and Use Cases. Java 8 brought a wave of powerful new features tha...
- Wednesday, January 15, 2025
An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples. Functional interfaces are an important concept in ...
- Wednesday, January 15, 2025
Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications. Spring Core provides the foundation for all other ...
- Wednesday, January 15, 2025
Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples. Java is a powerful and versatile programming langu...
- Monday, January 13, 2025
Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications. The Map interface in Java is a powerful and flexib...