Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples
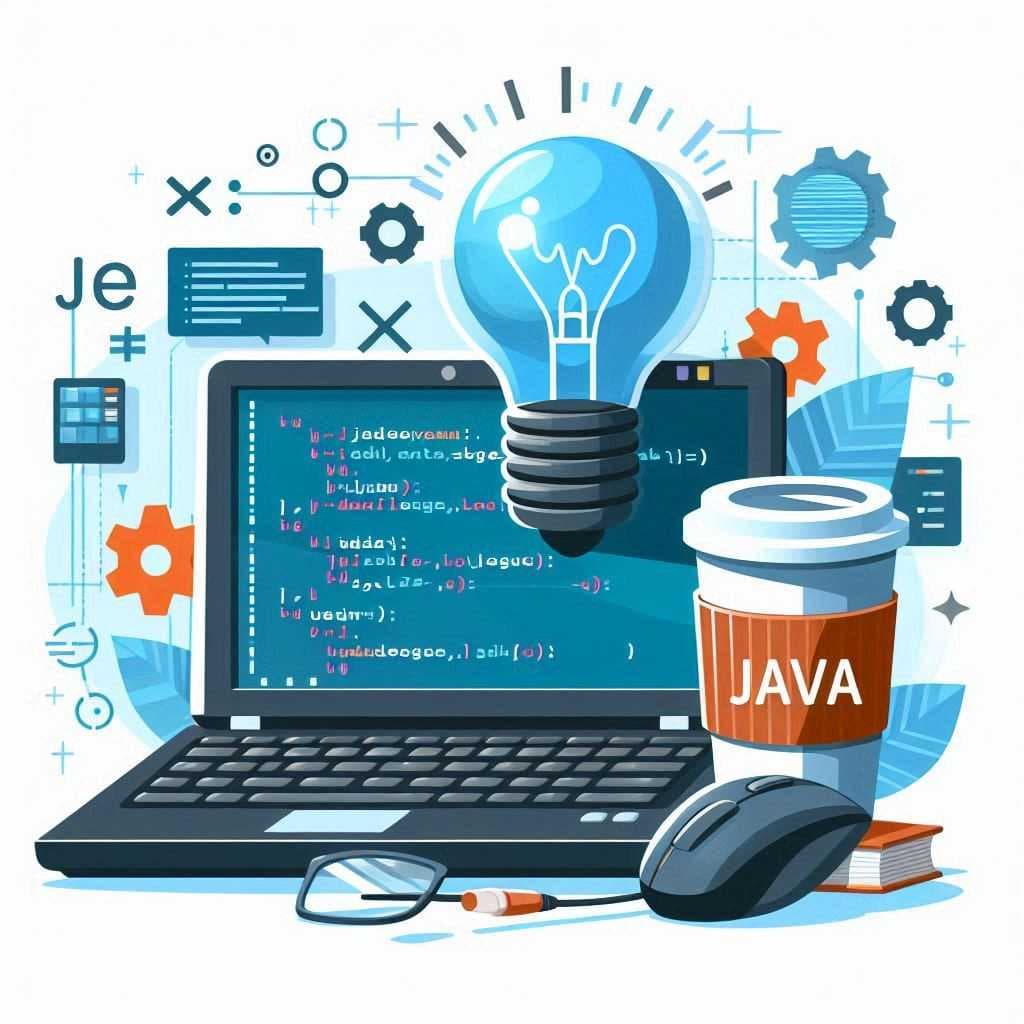
Java is a widely-used, beginner-friendly programming language. It supports object-oriented programming, making it ideal for creating robust, reusable, and scalable applications. Let’s explore its fundamentals in detail.
What is Java?
Java is a high-level, object-oriented, platform-independent programming language. It was developed by James Gosling in 1995 at Sun Microsystems and is now maintained by Oracle. Java’s slogan, "Write Once, Run Anywhere" (WORA), means compiled Java code can run on any device with a Java Virtual Machine (JVM).
Java is used in:
- Desktop Applications: Like IDEs, media players, etc.
- Web Applications: Frameworks like Spring Boot power many modern websites.
- Mobile Applications: Android apps are predominantly built using Java.
- Enterprise Systems: Large-scale backend systems in banks and insurance.
Why Learn Java?
- Easy to Learn: Its simple syntax is easy to understand for beginners.
- Highly Versatile: From mobile apps to web servers, Java is used everywhere.
- High Demand: Many organizations look for Java developers, offering high-paying jobs.
- Community Support: Java has an extensive online community for learning and problem-solving.
Setting Up Java
To write and run Java programs, you need the Java Development Kit (JDK) and optionally an Integrated Development Environment (IDE) for better coding experiences.
Step 1: Install JDK
- Download from the Oracle website.
- Follow the installation instructions for your operating system.
Step 2: Verify Installation
After installation, open your terminal or command prompt and type:
java -version
You should see the installed Java version.
Step 3: Install an IDE
Popular IDEs include:
- Eclipse: Easy-to-use and beginner-friendly.
- IntelliJ IDEA: Advanced tools for experienced developers.
- VS Code: Lightweight and extensible with plugins.
Writing Your First Java Program
Code:
// Simple Java program to print "Hello, World!"
public class HelloWorld { // Class declaration
public static void main(String[] args) { // Main method, the entry point
System.out.println("Hello, World!"); // Prints text to the console
}
}
Explanation:
public class HelloWorld
: Declares a class namedHelloWorld
.public static void main(String[] args)
: This is the starting point of any Java program. Themain
method runs when the program is executed.System.out.println()
: Prints text followed by a new line.
Steps to Run:
- Save the file as
HelloWorld.java
. - Open the terminal, navigate to the file directory, and compile it:
This generates a file namedjavac HelloWorld.java
HelloWorld.class
. - Run the program:
Output:java HelloWorld
Hello, World!
Variables and Data Types
A variable is a container for storing data. Java variables must have a type that determines the kind of data they store.
Primitive Data Types:
int
: Stores whole numbers (e.g., -100, 0, 50).
Example:int age = 25;
float
anddouble
: Store decimal numbers.
Example:double salary = 45000.75; float height = 5.9f; // Float requires an 'f' suffix
char
: Stores single characters.
Example:char grade = 'A';
boolean
: Stores true or false values.
Example:boolean isEligible = true;
Non-Primitive Data Types:
String
: Stores sequences of characters.
Example:String name = "Alice";
- Arrays: Used to store multiple values of the same type. (Explained later in detail.)
Arrays in Java
An array is a collection of elements of the same type stored in contiguous memory locations. Arrays are used when you need to work with multiple values together.
Example 1: Declaring and Initializing an Array
public class ArrayExample {
public static void main(String[] args) {
int[] numbers = {10, 20, 30, 40, 50}; // Declare and initialize an array
for (int i = 0; i < numbers.length; i++) { // Loop through the array
System.out.println("Element at index " + i + ": " + numbers[i]);
}
}
}
Explanation:
- Declaration:
int[] numbers
creates an array to hold integers. - Initialization:
{10, 20, 30, 40, 50}
assigns values to the array. - Accessing Elements: Use
numbers[i]
to access the element at indexi
. - Length:
numbers.length
gives the size of the array.
Output:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
Example 2: Multidimensional Array
public class MultiArrayExample {
public static void main(String[] args) {
int[][] matrix = { {1, 2}, {3, 4}, {5, 6} }; // 2D array
for (int i = 0; i < matrix.length; i++) { // Rows
for (int j = 0; j < matrix[i].length; j++) { // Columns
System.out.print(matrix[i][j] + " ");
}
System.out.println(); // New line after each row
}
}
}
Explanation:
- 2D Array:
int[][] matrix
represents a table-like structure. - Nested Loops: Iterate over rows and columns.
- Accessing Elements: Use
matrix[i][j]
to access elements.
Output:
1 2
3 4
5 6
Control Statements
Control statements determine the flow of program execution.
If-Else Example:
public class IfElseExample {
public static void main(String[] args) {
int number = -5;
if (number > 0) {
System.out.println("Positive number");
} else {
System.out.println("Non-positive number");
}
}
}
For Loop Example:
public class ForLoopExample {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) { // Loop from 1 to 5
System.out.println("Count: " + i);
}
}
}
Object-Oriented Programming (OOP)
OOP is a programming paradigm based on objects, which are instances of classes.
Example: Creating and Using Classes
public class Car {
String brand;
int speed;
void displayDetails() {
System.out.println("Brand: " + brand);
System.out.println("Speed: " + speed);
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car(); // Create an object
car.brand = "Toyota"; // Set properties
car.speed = 180;
car.displayDetails(); // Call the method
}
}
Output:
Brand: Toyota
Speed: 180
Conclusion :
Java is a powerful and versatile programming language, ideal for beginners and professionals alike. Its object-oriented approach, platform independence, and extensive community support make it a preferred choice for various applications. From basic syntax to advanced concepts like OOP and arrays, mastering Java provides a strong foundation for developing robust and scalable software. By practicing regularly and experimenting with examples, you can build your skills and become proficient in Java programming.
If something seems incorrect or needs adjustment, please let me know!
Comments
Blog Categories
Latest Blogs
- Thursday, January 16, 2025
A Complete Beginner’s Guide to Java Stream API with Detailed Examples. The Stream API in Java 8 simplifies data processin...
- Thursday, January 16, 2025
Java 8: A Comprehensive Guide with Examples and Use Cases. Java 8 brought a wave of powerful new features tha...
- Wednesday, January 15, 2025
An Excellent Beginner’s Guide to Understanding Functional Interfaces in Java with Simple Examples. Functional interfaces are an important concept in ...
- Wednesday, January 15, 2025
Mastering Spring Core: A Comprehensive and Detailed Guide for Java Developers to Build Robust, Scalable Applications. Spring Core provides the foundation for all other ...
- Wednesday, January 15, 2025
Comprehensive Beginner’s Guide to Java: Detailed Explanations for Every Concept with In-Depth Examples. Java is a powerful and versatile programming langu...
- Monday, January 13, 2025
Understanding the Map Interface in Java: Features, Implementations, and Real-World Applications. The Map interface in Java is a powerful and flexib...